Checkbox class – material library – Dart API
API docs for the Checkbox class from the material library, for the Dart programming language.
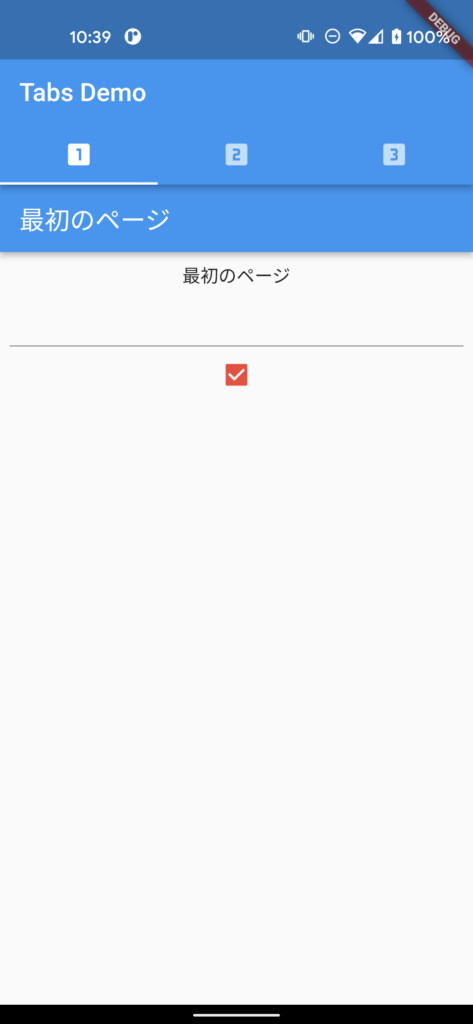
lib/my_first_page.dart
import 'package:flutter/material.dart'; import 'package:flutter_gen/gen_l10n/app_localizations.dart'; class MyFirstPage extends StatefulWidget { const MyFirstPage({Key? key}) : super(key: key); @override State<MyFirstPage> createState() => _MyFirstPageState(); } class _MyFirstPageState extends State<MyFirstPage> { late TextEditingController _controller; bool isChecked = false; @override void initState() { super.initState(); _controller = TextEditingController(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { Color getColor(Set<MaterialState> states) { const Set<MaterialState> interactiveStates = <MaterialState>{ MaterialState.pressed, MaterialState.hovered, MaterialState.focused, }; if (states.any(interactiveStates.contains)) { return Colors.blue; } return Colors.red; } return Scaffold( appBar: AppBar( title: Text(AppLocalizations.of(context)!.firstPage), ), body: Container( padding: const EdgeInsets.all(8), child: Column( children: <Widget>[ Text(AppLocalizations.of(context)!.firstPage), TextField( controller: _controller, onSubmitted: (String value) async { await showDialog<void>( context: context, builder: (BuildContext context) { return AlertDialog( title: const Text('Title'), content: Text( '"$value", which has length ${value.characters.length}.'), actions: <Widget>[ TextButton( onPressed: () { Navigator.pop(context); }, child: const Text('OK'), ), ], ); }, ); }, ), Checkbox( checkColor: Colors.white, fillColor: MaterialStateProperty.resolveWith(getColor), value: isChecked, onChanged: (bool? value) { setState(() { isChecked = value!; }); }), ], ), ), ); } }